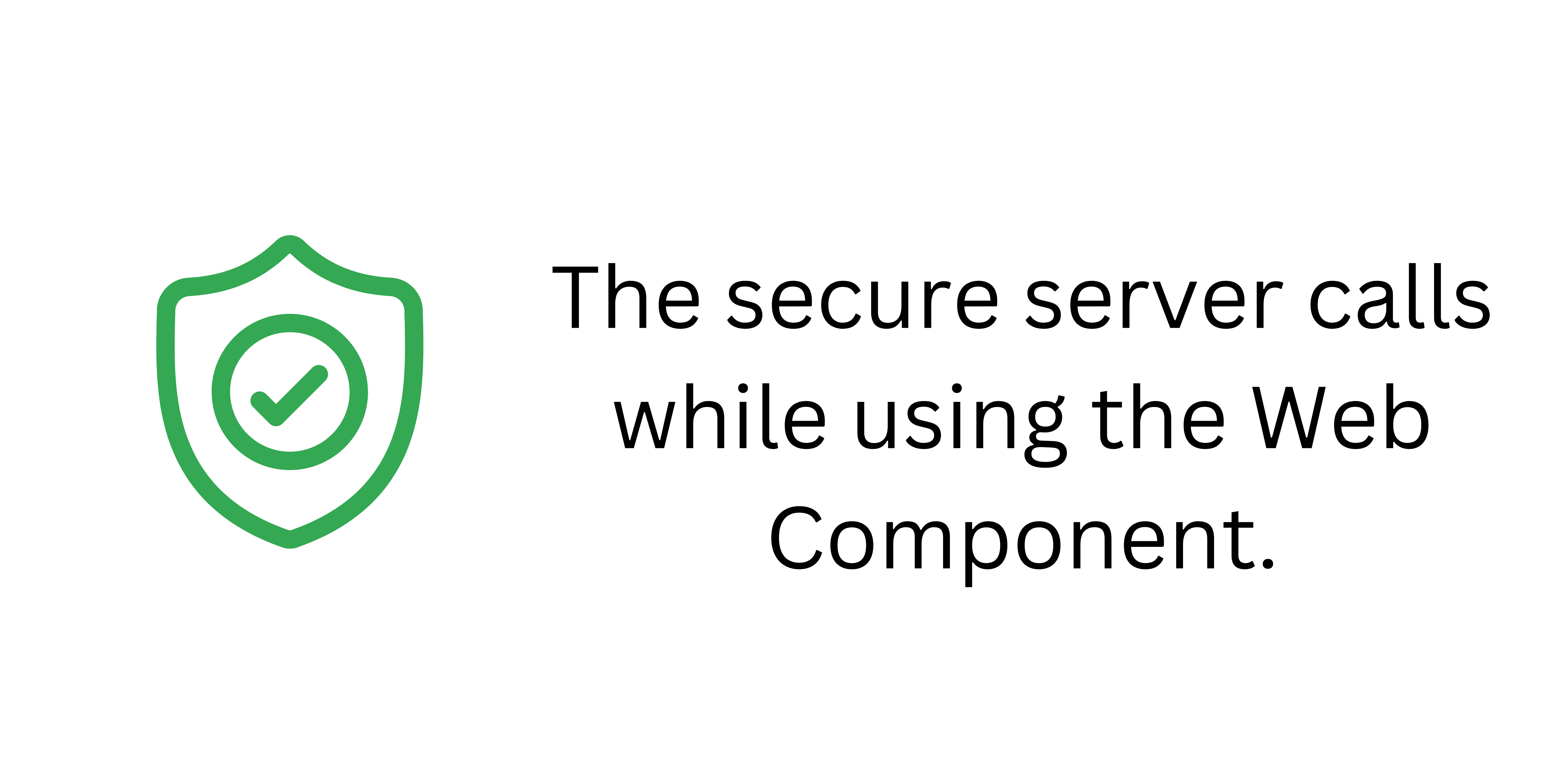
Most app developers use a cloud database or some sort of server to call data from when the application is live. Cloud databases add more power to apps by serving fresh and new information each time a user uses the app.
While creating an app on builders like Niotron, MIT App Inventor, and Kodular. A developer uses Web components for API calls. Using these APIs data is CRUD from the server. But here comes the security issue. Most of the time a developer doesn’t use any client authentication method on the server. And hence sometimes attackers use the same API URLs to update or destroy the data stored on the server.
So this blog is all about include a basic client authentication on server.
Server Configuration
The server code is PHP as we are using a PHP server for this example. In this, we are going to include 3 layers of security.
- User-Agent, the first check is of User-Agent to check if the client request is coming from Web Component or from an external browser. For this server code checks if the User-Agent is DALVIK if not the It cut off the further process.
- The region, the second check is of X-Region which tells the server about the time zone of the client request for further use. By default, it allows every time zone. But a developer can easily restrict a particular time zone anytime from the server.
- Authorization Token, This is the third but most important in this entire authentication process. Header token. In our app, we wrote some blocks to generate this token. It is a Client auth token. When a request arrives on the server. The server generates a new Server token of the request and then compares it with the client token. If the token matches, then the server responds with a success message.
Please note that, In this example we are not going to store or get any data from server. This is just and example of using auth token in app to make secured server calls. And in response server sends JSON data including Status and message. But you are free to modify it for your use.
Server Codes
Create a new file ‘DIY-security.php’ in the public directory of your hosting server. Note, you are free to use any name of the file. Get code from my GitHub repo.
<?php
$SecretKey = "Niotron";
$headers = getallheaders();
/*Default Response*/
$response = array("status"=>false,"msg"=>"unknown device location");
/*Get Client time zone*/
$timeZone = (isset($headers["X-Region"]) && $headers["X-Region"] !== "")? $headers["X-Region"] : "Asia/Kolkata";
$zoneList = timezone_identifiers_list();
/*Check User Agent*/
$response["msg"] = "The client request is not from app.";
if(isset($headers["User-Agent"]) && strpos($headers["User-Agent"], 'Dalvik') !== false){
if(in_array($timeZone, $zoneList)){
/*Change default time zone*/
date_default_timezone_set($timeZone);
$time = time();
$response["msg"] = "invalid credentials, check and try again.";
/*check for auth token*/
if(isset($headers["Authorization"]) && $headers["Authorization"] !== ""){
$token = str_replace("Basic ","", $headers["Authorization"]);
$decoded_token = base64_decode($token);
/*Validate decoded token*/
$Str1 = preg_replace('/[\x00-\x1F\x7F-\xFF]/', '', $decoded_token);
if($Str1!=$decoded_token || $Str1 == ''){
$response["msg"] = "invalid or broken token, try again.";
die(json_encode($response));
}
/*Get Index*/
$index = substr($decoded_token,(strlen($decoded_token) -2),2);
/*Generate Server Token*/
$serverToken = sha1($index . date("j/m/Y", $time) . $SecretKey . date("h:i", $time)). $index;
/*Compare Token*/
if($decoded_token !== $serverToken){
$response["msg"] = "invalid token, try again.";
die(json_encode($response));
}
/*Success- Do any action and return respose.*/
$response["status"] = true;
$response["msg"] = "Token Verified Successfully";
}
}
}
echo json_encode($response);
?>
Client Configuration
On client side, few builder specific components are enough. We are going to use,
- Button, to trigger action
- Label, to display server response
- Security component, to generate the hash of the data.
- Clock, to get the current time of the device.
- Device info, to get Device TimeZone
- Web Component, to make server calls.
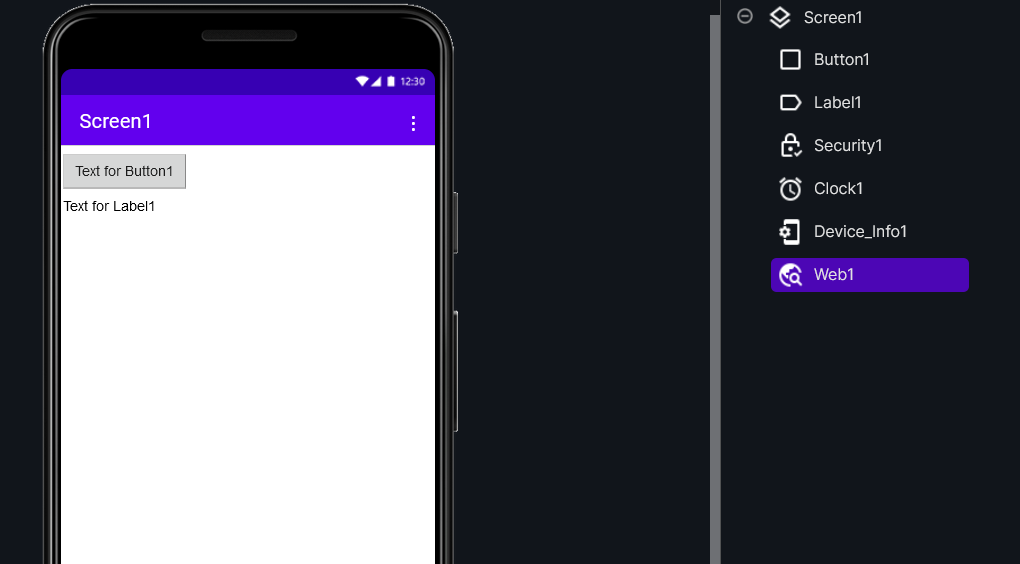
Blocks Coding
Total no of blocks : 39, Feel free to use my API url for testing.
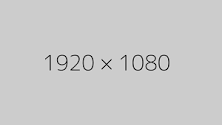
Use this basic securoty authentication method, and leave your valuable feedback in the comments below. Also if you have any suggestion on this security method do let me know.
Note, I’m not claiming that the server calls performed by using this basic auth token technique will completely secure your API calls. Security is a very big topic, you have to keep an eye on attacks performed using your API URLs and continuously learning and improving them. If you need any help related to the security of API calls. Feel free to contact me.